목차
1. 예외 종류
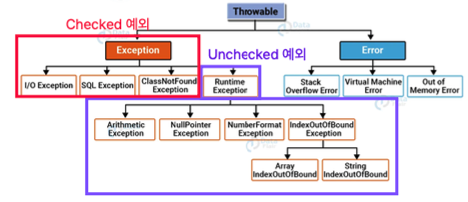
- Checked 예외
- 컴파일 시점에 체크 ⇒ 컴파일 에러가 가장 안전한 에러임!
- 명시적인 예외 처리 필요
- Unchecked 예외
- 런타임 시점에 체크 ⇒ 다소 위험함
- 명시적인 예외 처리 강제 x
1-1. 대표적인 예외
- Checked 예외
- IOException
- 입출력 작업 중에 문제가 생겼을 때 발생
ex) 파일을 읽거나 쓸 때 파일 시스템에 접근할 수 없거나 네트워크 연결이 끊어졌을 때
- 입출력 작업 중에 문제가 생겼을 때 발생
- FileNotFoundException
- 특정 파일을 찾을 수 없을 때 발생
ex) 파일 경로가 잘못되었거나, 파일이 존재하지 않을 때
- 특정 파일을 찾을 수 없을 때 발생
- ClassNotFoundException
- 특정 클래스를 찾을 수 없을 때 발생
ex) Class.forName() 메서드를 사용하여 클래스를 동적으로 로드할 때 클래스 경로에 해당 클래스가 존재하지 않을 때
- 특정 클래스를 찾을 수 없을 때 발생
- SQLException
- 데이터베이스 작업 중에 문제가 발생할 때 발생
ex) SQL 쿼리의 구문 오류, DB 연결 문제, 트랜잭션 처리 중 오류 등
- 데이터베이스 작업 중에 문제가 발생할 때 발생
- NoSuchMethodException
- 리플렉션을 사용하여 특정 메서드를 찾을 때 해당 메서드가 존재하지 않을 때 발생
- IOException
- Unchecked 예외
- NullPointerException
- 객체 참조가 없는 상태, 즉 null 값을 갖는 참조 변수로 객체 접근 연산자인 . (dot) 사용했을 때 발생
- ArrayIndexOutOfBoundsException
- 인덱스 범위를 벗어나게 배열에 접근 시 발생
- IllegalArgumentException
- 메서드에 잘못된 인자를 전달했을 때 발생
ex) 메서드가 요구하는 특정 범위의 값을 넘어선 값을 인자로 전달한 경우
- 메서드에 잘못된 인자를 전달했을 때 발생
- ArithmeticException
- 산술 연산 중에 문제가 발생했을 때 발생
ex) 어떤 수를 0으로 나눌 때
- 산술 연산 중에 문제가 발생했을 때 발생
- NumberFormatException
- Wrapper 클래스 (Integer, Double emd)의 정적 메서드인 parseXXX() 메서드 사용 시
숫자로 변환할 수 없는 문자열이 매개변수로 들어올 때 발생
ex)int value2 = Integer.parseInt("a100");
- Wrapper 클래스 (Integer, Double emd)의 정적 메서드인 parseXXX() 메서드 사용 시
- ClassCastException
- 변환할 수 없는 타입으로 캐스팅 시도 시 발생
ex) Animal animal = new Dog();
Cat cat = (Cat) animal;
- 변환할 수 없는 타입으로 캐스팅 시도 시 발생
- NullPointerException
2. 예외 처리
2-1. try-catch
- 예외 발생 가능한 코드를 try 블록 안에 작성
- 발생한 예외 처리 코드를 catch 블록 안에 작성
- catch 문 ⇒ 상위 클래스 (ex. Exception)이 상단 catch 문에 존재하면 x !! ⇒ 하위 클래스 부터 상단에 오게끔 배치
try {
// 예외 발생 가능 코드
} catch (ExceptionType1 e) {
// ExceptionType1 처리 코드
} catch (ExceptionType2 e) {
// ExceptionType2 처리 코드
}
// ExceptionType2가 ExeptionType1 보다 상위 클래스 여야 함!
2-2. try-catch-finally
- finally 블록 : 예외 발생 여부와 상관없이 실행되는 코드
- 주로 자원 해제와 같은 정리 작업에 활용
try {
// 예외 발생 가능 코드
} catch (Exception e) {
// Exception 처리 코드
} finally {
// 예외 발생 여부와 상관없이 실행될 코드
}
2-3. try-with-resources
- 자동 자원 반환을 지원 (AutoClosable 인터페이스 구현 객체) ⇒ finally x (자동 자원 반환)
try(Resource resource = new Resource() // 사용할 리소스 ) {
// 예외 발생 가능 코드
} catch (Exception e) {
// 예외 처리
}
- 조건 : 리소스 객체는 java.lang.AutoCloseable 인터페이스를 구현해야 함
public class FileInputStream implements AutoCloseable {
private String file;
public FileInputStream(String file) {
this.file = file;
}
public void read() {
System.out.println("Read " + file);
}
@Override
public void close() throws Exception {
System.out.println(file + " is closed.");
}
}
public class TryWithResourcesExample {
public static void main(String[] args) {
try(FileInputStream fis = new FileInputStream("file.txt")) {
fis.read();
throw new Exception();
} catch (Exception e) {
System.out.println("Exception processing code executed.");
}
}
}
/* 실행결과
Read file.txt
file.txt is closed.
Exception processing code executed. */
⇒ 예외가 발생하는 즉시 자동으로 FileInputStream의 close()가 호출됨!
2-4. 멀티 catch
- 자바 7부터 사용 가능
- 하나의 catch 블록에서 여러 개의 exception 처리
- catch(Exception1 | Exception2 | … )
try {
String data1 = args[0];
String data2 = args[1];
int value1 = Integer.parseInt(data1);
int value2 = Integer.parseInt(data2);
int result = value1 + value2;
System.out.println(data1 + " + " + data2 + " = " + result);
} catch(ArrayIndexOutOfBoundsException | NumberFormatException e) {
System.out.println("Arguments are not enough. Or can not change a variable into integer.");
} catch(Exception e) {
System.out.println("There is a problem for execution.");
} finally {
System.out.println("Do it again.");
}
3. 예외 처리 throws
- 현재 위치에서 예외 처리 x, 호출한 곳으로 예외 처리를 던짐(전가시킴)
- 메서드 선언부에 사용
public void someMethod() throws IOException, SQLException {
// 메서드 구현
}
4. 좋은 예외 처리 방법
1. 구체적인 예외 처리 우선 (하위 클래스 먼저 배치)
2. 필요 이상의 예외는 던지지 x
3. 예외 메시지에 오류 분석에 도움이 되는 정보 포함
4. 공통 예외 처리 로직은 별도 메서드로 분리
5. 자원 사용하는 경우 finally 불록에서 자원 해제 or try-with-resources 사용 권장
'Java' 카테고리의 다른 글
[Java] 빌더 패턴 (+ @Builder) (0) | 2024.09.15 |
---|---|
[Java] 자바 제네릭스 (Generics) (0) | 2024.07.28 |
[Java] 입출력(I/O), 스트림, 버퍼 (0) | 2024.07.28 |
[Java] 자바 메모리 구조 (0) | 2024.07.09 |
[Java] final (0) | 2024.07.09 |
1. 예외 종류
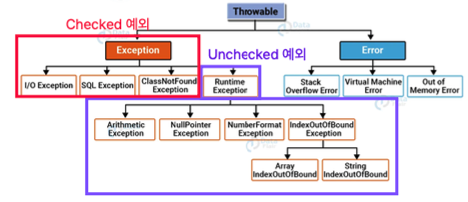
- Checked 예외
- 컴파일 시점에 체크 ⇒ 컴파일 에러가 가장 안전한 에러임!
- 명시적인 예외 처리 필요
- Unchecked 예외
- 런타임 시점에 체크 ⇒ 다소 위험함
- 명시적인 예외 처리 강제 x
1-1. 대표적인 예외
- Checked 예외
- IOException
- 입출력 작업 중에 문제가 생겼을 때 발생
ex) 파일을 읽거나 쓸 때 파일 시스템에 접근할 수 없거나 네트워크 연결이 끊어졌을 때
- 입출력 작업 중에 문제가 생겼을 때 발생
- FileNotFoundException
- 특정 파일을 찾을 수 없을 때 발생
ex) 파일 경로가 잘못되었거나, 파일이 존재하지 않을 때
- 특정 파일을 찾을 수 없을 때 발생
- ClassNotFoundException
- 특정 클래스를 찾을 수 없을 때 발생
ex) Class.forName() 메서드를 사용하여 클래스를 동적으로 로드할 때 클래스 경로에 해당 클래스가 존재하지 않을 때
- 특정 클래스를 찾을 수 없을 때 발생
- SQLException
- 데이터베이스 작업 중에 문제가 발생할 때 발생
ex) SQL 쿼리의 구문 오류, DB 연결 문제, 트랜잭션 처리 중 오류 등
- 데이터베이스 작업 중에 문제가 발생할 때 발생
- NoSuchMethodException
- 리플렉션을 사용하여 특정 메서드를 찾을 때 해당 메서드가 존재하지 않을 때 발생
- IOException
- Unchecked 예외
- NullPointerException
- 객체 참조가 없는 상태, 즉 null 값을 갖는 참조 변수로 객체 접근 연산자인 . (dot) 사용했을 때 발생
- ArrayIndexOutOfBoundsException
- 인덱스 범위를 벗어나게 배열에 접근 시 발생
- IllegalArgumentException
- 메서드에 잘못된 인자를 전달했을 때 발생
ex) 메서드가 요구하는 특정 범위의 값을 넘어선 값을 인자로 전달한 경우
- 메서드에 잘못된 인자를 전달했을 때 발생
- ArithmeticException
- 산술 연산 중에 문제가 발생했을 때 발생
ex) 어떤 수를 0으로 나눌 때
- 산술 연산 중에 문제가 발생했을 때 발생
- NumberFormatException
- Wrapper 클래스 (Integer, Double emd)의 정적 메서드인 parseXXX() 메서드 사용 시
숫자로 변환할 수 없는 문자열이 매개변수로 들어올 때 발생
ex)int value2 = Integer.parseInt("a100");
- Wrapper 클래스 (Integer, Double emd)의 정적 메서드인 parseXXX() 메서드 사용 시
- ClassCastException
- 변환할 수 없는 타입으로 캐스팅 시도 시 발생
ex) Animal animal = new Dog();
Cat cat = (Cat) animal;
- 변환할 수 없는 타입으로 캐스팅 시도 시 발생
- NullPointerException
2. 예외 처리
2-1. try-catch
- 예외 발생 가능한 코드를 try 블록 안에 작성
- 발생한 예외 처리 코드를 catch 블록 안에 작성
- catch 문 ⇒ 상위 클래스 (ex. Exception)이 상단 catch 문에 존재하면 x !! ⇒ 하위 클래스 부터 상단에 오게끔 배치
try {
// 예외 발생 가능 코드
} catch (ExceptionType1 e) {
// ExceptionType1 처리 코드
} catch (ExceptionType2 e) {
// ExceptionType2 처리 코드
}
// ExceptionType2가 ExeptionType1 보다 상위 클래스 여야 함!
2-2. try-catch-finally
- finally 블록 : 예외 발생 여부와 상관없이 실행되는 코드
- 주로 자원 해제와 같은 정리 작업에 활용
try {
// 예외 발생 가능 코드
} catch (Exception e) {
// Exception 처리 코드
} finally {
// 예외 발생 여부와 상관없이 실행될 코드
}
2-3. try-with-resources
- 자동 자원 반환을 지원 (AutoClosable 인터페이스 구현 객체) ⇒ finally x (자동 자원 반환)
try(Resource resource = new Resource() // 사용할 리소스 ) {
// 예외 발생 가능 코드
} catch (Exception e) {
// 예외 처리
}
- 조건 : 리소스 객체는 java.lang.AutoCloseable 인터페이스를 구현해야 함
public class FileInputStream implements AutoCloseable {
private String file;
public FileInputStream(String file) {
this.file = file;
}
public void read() {
System.out.println("Read " + file);
}
@Override
public void close() throws Exception {
System.out.println(file + " is closed.");
}
}
public class TryWithResourcesExample {
public static void main(String[] args) {
try(FileInputStream fis = new FileInputStream("file.txt")) {
fis.read();
throw new Exception();
} catch (Exception e) {
System.out.println("Exception processing code executed.");
}
}
}
/* 실행결과
Read file.txt
file.txt is closed.
Exception processing code executed. */
⇒ 예외가 발생하는 즉시 자동으로 FileInputStream의 close()가 호출됨!
2-4. 멀티 catch
- 자바 7부터 사용 가능
- 하나의 catch 블록에서 여러 개의 exception 처리
- catch(Exception1 | Exception2 | … )
try {
String data1 = args[0];
String data2 = args[1];
int value1 = Integer.parseInt(data1);
int value2 = Integer.parseInt(data2);
int result = value1 + value2;
System.out.println(data1 + " + " + data2 + " = " + result);
} catch(ArrayIndexOutOfBoundsException | NumberFormatException e) {
System.out.println("Arguments are not enough. Or can not change a variable into integer.");
} catch(Exception e) {
System.out.println("There is a problem for execution.");
} finally {
System.out.println("Do it again.");
}
3. 예외 처리 throws
- 현재 위치에서 예외 처리 x, 호출한 곳으로 예외 처리를 던짐(전가시킴)
- 메서드 선언부에 사용
public void someMethod() throws IOException, SQLException {
// 메서드 구현
}
4. 좋은 예외 처리 방법
1. 구체적인 예외 처리 우선 (하위 클래스 먼저 배치)
2. 필요 이상의 예외는 던지지 x
3. 예외 메시지에 오류 분석에 도움이 되는 정보 포함
4. 공통 예외 처리 로직은 별도 메서드로 분리
5. 자원 사용하는 경우 finally 불록에서 자원 해제 or try-with-resources 사용 권장
'Java' 카테고리의 다른 글
[Java] 빌더 패턴 (+ @Builder) (0) | 2024.09.15 |
---|---|
[Java] 자바 제네릭스 (Generics) (0) | 2024.07.28 |
[Java] 입출력(I/O), 스트림, 버퍼 (0) | 2024.07.28 |
[Java] 자바 메모리 구조 (0) | 2024.07.09 |
[Java] final (0) | 2024.07.09 |